What is the CSS :nth-child() selector?
:nth-child() is a CSS pseudo-class that selects elements based on their position in a collection of sibling elements. The selected positions are determined by an integer, keyword, or expression located inside the pseudo-class' parentheses.
CSS :nth-child() Syntax
As a pseudo-class, nth-child is preceded by a colon and followed by a set of parentheses. Inside the parentheses we specify an argument, this argument represents which sibling child(ren) we want to select.
Syntax Breakdown
- Before the colon we can write a selector for the element(s) that the nth-child must match.
- Inside the parentheses (argument) we write which sibling child(ren) we want to select
- We do this with an integer, an expression, or a keyword
Integer
When you're only specifying an integer, you're selecting a single element per group.
Integer Example
If you want to select the third list item, you can do so by simply inputting a 3 inside the nth-child's parentheses.
HTML
CSS
Output
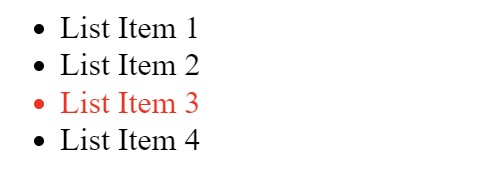
Integer Example 2
List items are naturally grouped together inside ordered, or unordered lists. When we specify that we want the second list-item selected, it selects the second list item in both lists.
HTML
CSS
Output
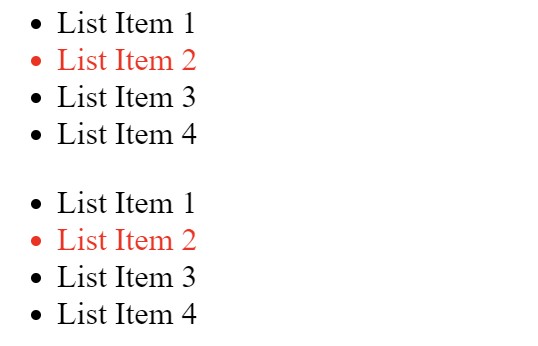
Keywords
The keywords even and odd can be specified as our nth-child's argument. The even keyword selects the sibling child(ren) that are in even positions in their group (2nd, 4th, 6th, etc.). The odd keyword selects the sibling child(ren) that are in odd positions in their group (1st, 3rd, 5th, etc.).
Keywords Example 1
In this example, we have a single ordered list <ol> and we'd like to change all the even list items (2, 4, 6, etc.) to have red text. We can simply use the keyword even inside out nth-child's parentheses
HTML
CSS
Output

Keywords Example 2
Like the first keywords example, we'd like to select all the even list item elements, but this time we have two ordered lists <ol>. As you can see, by using nth-child(even) it changes all the even (2, 4, 6, etc.) list items' text color to red.
HTML
CSS
Output
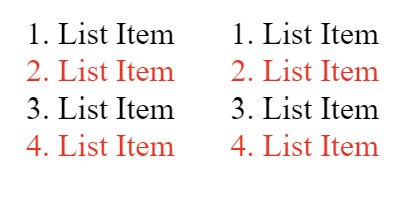
Expression
As an alternative to using an integer, or a keyword, we can use the expression an or an + b. Using an expression allows us to make more advanced selections.
To breakdown the expression an + b:
- a is an integer
- n starts at 0 and iterates up by 1
- + is an optional operator (you can use the - operator)
- b is an optional integer that accompanies the optional operator
Expression Example 1
In this example, we're using the expression an - specifically 2n. This selects every second paragraph inside our "paragraphs" div.
HTML
CSS
Expression Explanation
Output
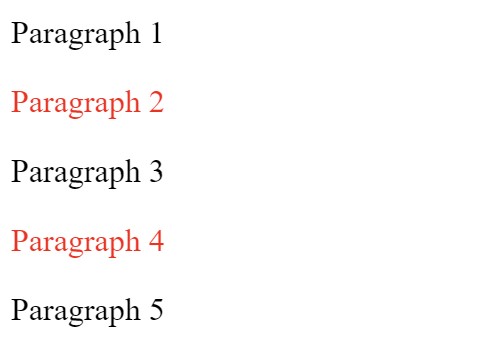
Expression Example 2
In this example, we're using the expression an + b - specifically 2n + 1. This selects every second paragraph inside our "paragraphs" div, starting with the first paragraph.
HTML
CSS
Expression Explanation
Output

Expressions Example 3
In this example we're using the expression an + b again, but we're using a negative n value, specifically -n + 3.
HTML
CSS
Expression Explanation
Output

Video Tutorial
Also available on YouTube and Instagram.
CSS :nth-child() Examples
The following examples are aimed to help you see how nth-child can be used in real-world scenarios.
Example 1 - Style Navbar Elements Differently
Example 1 Scenario
Your garden center website sells some items online and some items in-store. You'd like to host a sales event where all the items that you can purchase online are discounted, as long as they are not already on clearance pricing. The sidebar on the store lists links to all the different categories of products. The first three links are always clearance pricing, the rest of the links are never clearance pricing, but some of them are for in-store only. You'd like to highlight the fact that there is a sale on particular items by changing the appropriate links' text color to red.
Thinking This Through
- We never want to select the first three list items
- After the first three items only some of them should be selected based on if they're leading to products sold online
- There may be other lists present in the product page for descriptions and other things, so we need to ensure our selection mechanism only selects the list in the sidebar
- We'll use the class ecomm to identify list items that can be purchased online
Example 1 Solution
HTML
CSS
Expression Explanation
Using the calculations above, it shows that our expression (n + 4) is indeed skipping the first three elements - avoiding the items that have clearance pricing. But it also appears that it will start selecting all the items past the first three, even ones that are only sold in-store.
This is where the rest of the selector comes in...
Output
Example 2 - Dynamic Festive Styles
Example 2 Scenario
Your client's website has a collection of 4 content boxes on the home page. Each content box contains a heading, a paragraph, and a button. During the holiday season, your client would like every other button to be changed to green, with the remainder changed to red.
Thinking This Through
- We'll have a wrapper div that will contain all of the content boxes, the wrapper div will have the class content-boxes
- To select every other content box we'll use two selectors and the keywords even and odd with our nth-child
Example 2 Solution
HTML
CSS
Output
Selector Explanations
10% Off Web Development Courses
Learn web development skills with interactive courses (free & paid) by Scrimba.
Using our link, get 10% off all Scrimba plans - click here!
Note: We receive a monetary kickback if you sign up using our link.
Sources
- nth-child MDN (Source)
- nth-child CSS-Tricks Almanac (Source)
- How nth-child Works (CSS-Tricks)